二叉树节点结构
1 2 3 4 5 6 7 8
| public class TreeNode{ int val; TreeNode left; TreeNode right; TreeNode(int x){ this.val = x; } }
|
前序遍历
前序遍历:根结点 —> 左子树 —> 右子树 (根节点在 前中后 那个位置 就叫什么遍历)
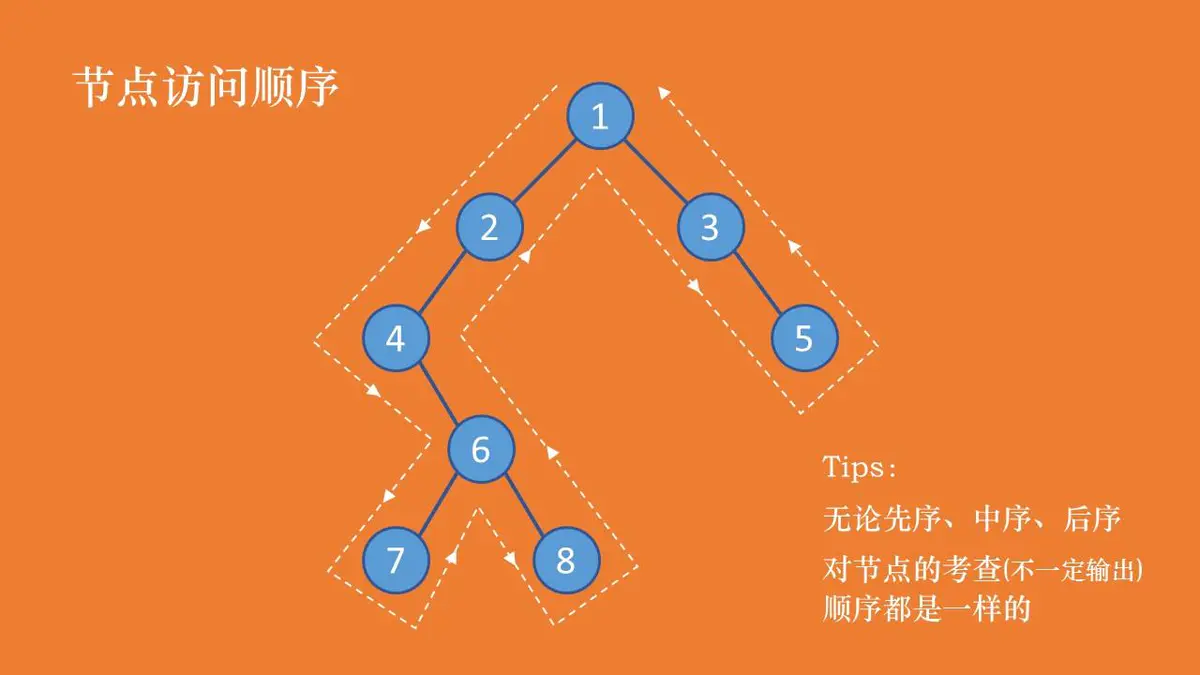
前序遍历:1 2 4 6 7 8 3 5
题目
144.二叉树的前序遍历
递归算法
1 2 3 4 5 6 7 8 9 10 11 12 13
| class Solution { List<Integer> res = new ArrayList<Integer>(); public List<Integer> preorderTraversal(TreeNode root){ if (root == null) return res; res.add(root.val); preorderTraversal(root.left); preorderTraversal(root.right); } return res; }
|
迭代法

1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18
| class Solution { public List<Integer> preorderTraversal(TreeNode root) { List<Integer> res = new ArrayList<Integer>(); Stack<TreeNode> stack = new Stack<TreeNode>(); while(root!=null || !stack.empty()){ while(root!=null){ res.add(root.val); stack.push(root); root = root.left; } root = stack.pop(); root = root.right; } return res; } }
|
中序遍历
中序遍历:左子树—> 根结点 —> 右子树 (根节点在 前中后 那个位置 就叫什么遍历)
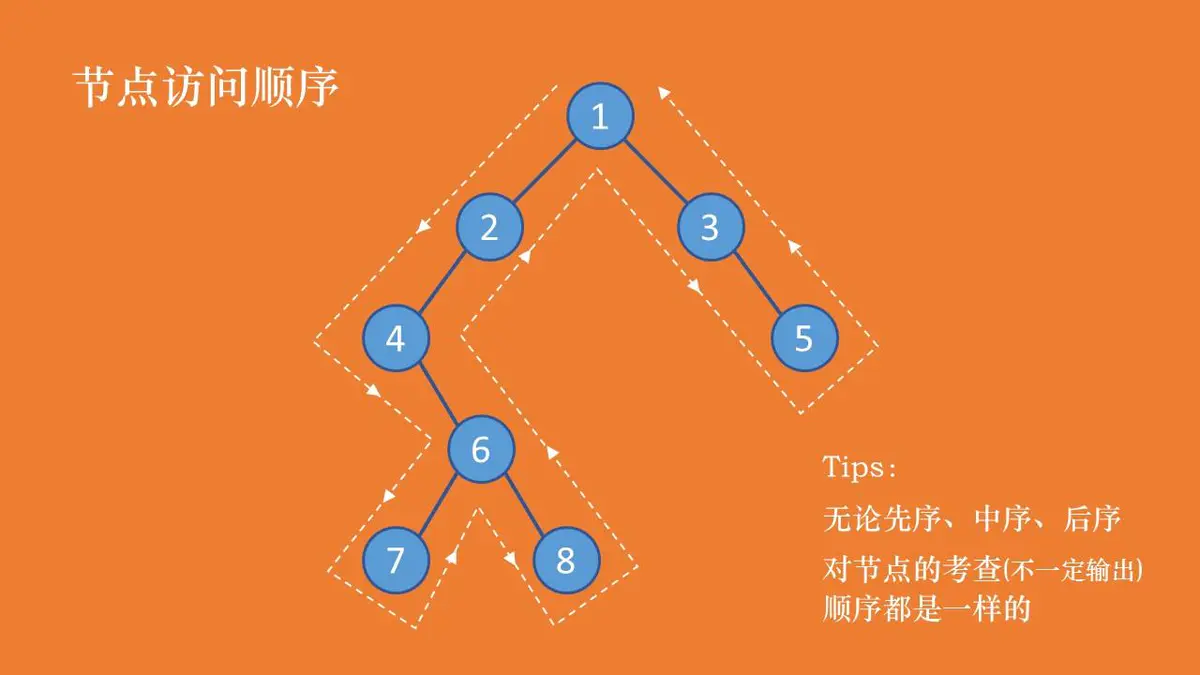
中序遍历 :4 7 6 8 2 1 3 5
题目
94.二叉树的中序遍历
递归算法
1 2 3 4 5 6 7 8 9 10
| class Solution { List<Integer> res = new ArrayList<Integer>(); public List<Integer> inorderTraversal(TreeNode root) { if(root == null) return res; inorderTraversal(root.left); res.add(root.val); inorderTraversal(root.right); return res; } }
|
迭代法

1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17
| class Solution { public List<Integer> preorderTraversal(TreeNode root) { List<Integer> res = new ArrayList<Integer>(); Stack<TreeNode> stack = new Stack<TreeNode>(); while(root!=null || !stack.empty()){ while(root!=null){ stack.push(root); root = root.left; } root = stack.pop(); res.add(root.val); root = root.right; } return res; } }
|
后续遍历
后序遍历: 左子树 —> 右子树 —>根结点 (根节点在 前中后 那个位置 就叫什么遍历)
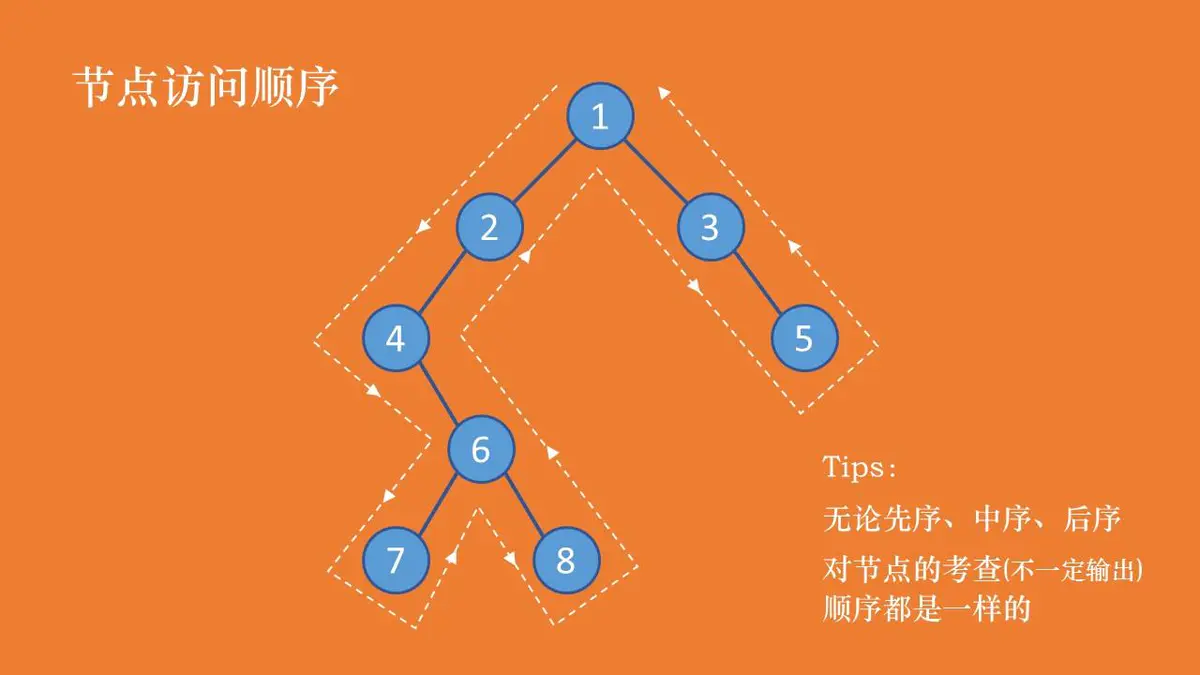
后序遍历:7 8 6 4 2 5 3 1
题目
145.二叉树的后序遍历
递归算法
后序遍历:左子树 —> 右子树 —> 根结点(根节点在 前中后 那个位置 就叫什么遍历)
1 2 3 4 5 6 7 8 9 10
| class Solution { List<Integer> res = new ArrayList<>(); public List<Integer> postorderTraversal(TreeNode root) { if (root == null) return res; postorderTraversal(root.left); postorderTraversal(root.right); res.add(root.val); return res; } }
|
迭代法
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23
| class Solution { public List<Integer> postorderTraversal(TreeNode root) { List<Integer> res = new ArrayList<Integer>(); Stack<TreeNode> stack = new Stack<>(); TreeNode pre = null; while(root!=null || !stack.empty()){ while(root!=null){ stack.push(root); root = root.left; } root = stack.peek(); if(root.right==null || root.right==pre){ res.add(root.val); pre = root; stack.pop(); root = null; }else{ root = root.right; } } return res; } }
|
第二种解法
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20
|
class Solution { public List<Integer> postorderTraversal(TreeNode root) { LinkedList res = new LinkedList(); Stack<TreeNode> stack = new Stack<>(); TreeNode pre = null; while(root!=null || !stack.empty()){ while(root!=null){ res.addFirst(root.val); stack.push(root); root = root.right; } root = stack.pop(); root = root.left; } return res; } }
|